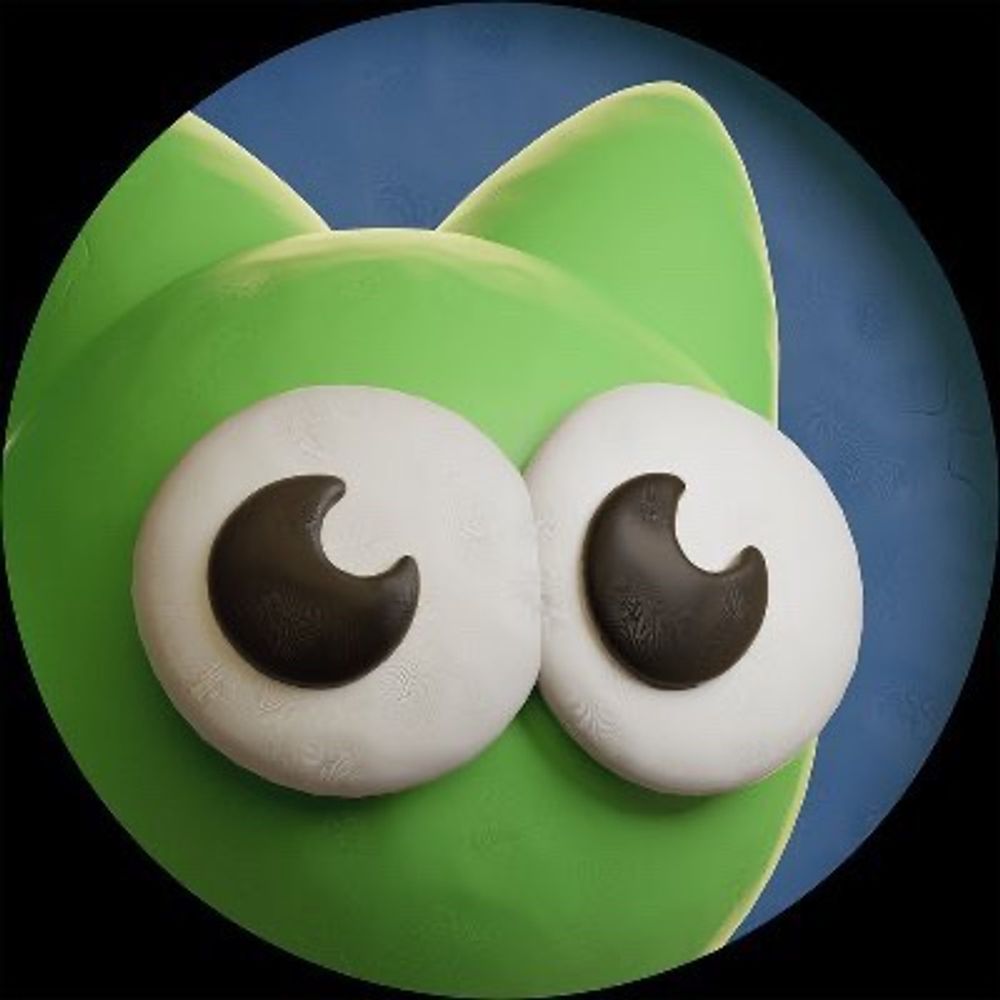
@jr3dful.bsky.social (JR3DFUL)
✨Shader Magic✨
UE5’s HLSL node is powerful, but inlining all the code gets messy, and standalone functions won’t work.
Structs organize logic into clean, reusable blocks, making shaders easier to manage.
Follow for more tips! 💚👀
#TechArt #GameDev #UnrealEngine #Shaders #3DArt #IndieDev
Posted at: 2025-03-25 15:00:07 UTC
Alt: // Blend color1 and color2 (50% blend), then blend the result with the average of all three colors
return lerp(lerp(color1, color2, 0.5), (color1 + color2 + color3) / 3.0, 0.5);
Alt: float3 Blend(float3 a, float3 b)
{
return lerp(a, b, 0.5);
}
float3 Average(float3 a, float3 b, float3 c)
{
return (a + b + c) / 3.0;
}
float3 Main(float3 a : COLOR1, float3 b : COLOR2, float3 c : COLOR3) : SV_Target0
{
return lerp(Blend(a, b), Average(a, b, c), 0.5);
}
return Main(color1, color2, color3);
Alt: struct Functions
{
float3 Blend(float3 a, float3 b)
{
return lerp(a, b, 0.5); // Blend 50% of each color
}
float3 Average(float3 a, float3 b, float3 c)
{
return (a + b + c) / 3.0; // Average of all three colors
}
float3 Main(float3 a : COLOR1, float3 b : COLOR2, float3 c : COLOR3) : SV_Target0
{
// Blend the result of Blend(a, b) and Average(a, b, c)
return lerp(Blend(a, b), Average(a, b, c), 0.5);
}
};
Functions f;
return f.Main(color1, color2, color3);